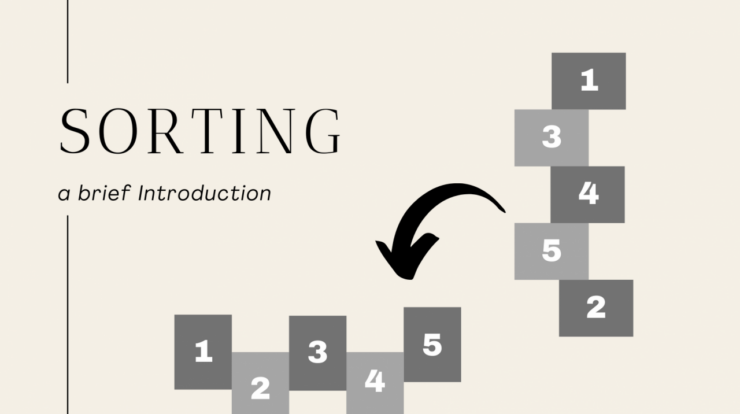
Sort definition – Sorting, a fundamental concept in computer science, plays a crucial role in organizing and manipulating data structures. From simple arrangements to complex algorithms, sorting empowers developers with the ability to efficiently retrieve and process information.
This comprehensive guide delves into the world of sorting, exploring its definition, types, applications, efficiency considerations, and advanced techniques. Get ready to embark on a journey that will illuminate the intricacies of sorting and its significance in data management.
1. Sort Definition
Sorting refers to the process of arranging data elements in a specific order, typically ascending or descending. It involves identifying and exchanging elements within a collection to achieve the desired order.
Sorting finds applications in numerous domains, including computer science, mathematics, and statistics. For instance, in data analysis, sorting helps identify patterns and trends by organizing data points in a meaningful way.
Examples of Sorting in Different Contexts
- Sorting numbers in ascending order to find the smallest or largest value
- Sorting names alphabetically to create a phone directory
- Sorting files by size to optimize storage space
2. Types of Sorting Algorithms
There are numerous sorting algorithms, each with its own approach and characteristics. Some common algorithms include:
- Bubble Sort:Iteratively compares adjacent elements and swaps them if they are out of order.
- Insertion Sort:Builds the sorted list one element at a time by inserting each unsorted element into its correct position.
- Merge Sort:Divides the input array into smaller subarrays, sorts them recursively, and then merges them back together.
Time and Space Complexities, Sort definition
The efficiency of a sorting algorithm is often measured in terms of its time and space complexities.
- Time Complexity:Measures the number of operations performed by the algorithm, typically expressed using Big O notation.
- Space Complexity:Measures the amount of additional memory required by the algorithm.
3. Applications of Sorting
Sorting has a wide range of applications in real-world scenarios, including:
- Data Analysis:Sorting helps identify patterns and trends by organizing data points in a meaningful way.
- Optimization:Sorting can be used to optimize resource allocation, scheduling, and other processes by identifying the best or worst elements.
- Decision-Making:Sorting can support decision-making by providing an organized view of data, making it easier to compare and evaluate options.
4. Efficiency Considerations
The efficiency of a sorting algorithm depends on several factors, including:
- Data Size:The number of elements to be sorted can significantly impact the sorting time.
- Data Type:The type of data being sorted (e.g., integers, strings) can affect the efficiency of comparison operations.
- Algorithm Choice:The choice of sorting algorithm can greatly influence the efficiency.
Techniques for Optimizing Sorting Algorithms
Several techniques can be employed to optimize sorting algorithms, such as:
- In-Place Sorting:Sorts the data without requiring additional memory space.
- Adaptive Sorting:Adjusts the sorting algorithm based on the characteristics of the input data.
- Parallel Sorting:Utilizes multiple processors or threads to improve sorting performance.
5. Visual Representation of Sorting
Sorting Algorithm | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n2) | O(1) |
Insertion Sort | O(n2) | O(1) |
Merge Sort | O(n log n) | O(n) |
Diagrams to Illustrate the Sorting Process
Various diagrams can be used to visualize the sorting process, such as:
- Bar Charts:Represent the data elements as bars, with the height of each bar indicating the value.
- Flowcharts:Illustrate the step-by-step process of the sorting algorithm.
6. Extensions of Sorting: Sort Definition
Advanced sorting techniques extend the capabilities of basic sorting algorithms:
- Radix Sort:Sorts data by individual digits or bits, making it efficient for large numbers.
- Bucket Sort:Divides the input data into buckets based on a range of values, making it suitable for non-uniformly distributed data.
Advantages and Disadvantages
These advanced techniques offer advantages and disadvantages:
- Radix Sort:Efficient for large numbers but requires additional memory space.
- Bucket Sort:Suitable for non-uniformly distributed data but may require manual tuning of bucket sizes.
Closure
Sorting stands as a cornerstone of data processing, providing a systematic approach to organizing and retrieving information. Its versatility extends across numerous fields, empowering us to make informed decisions, optimize processes, and gain valuable insights from complex datasets. As technology continues to advance, sorting algorithms will undoubtedly remain indispensable tools in the quest for efficient and accurate data management.
Answers to Common Questions
What is the primary goal of sorting?
Sorting aims to rearrange elements in a data structure according to a specific order, making it easier to search, retrieve, and process the data.
Can sorting be applied to different data types?
Yes, sorting algorithms can be tailored to work with various data types, including numbers, strings, objects, and even custom data structures.
What factors influence the efficiency of sorting algorithms?
The efficiency of sorting algorithms is primarily determined by the size of the input data, the complexity of the algorithm itself, and the hardware capabilities.